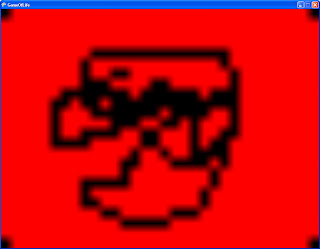
Removing that doggone blending is actually quite simple. You have to modify your spriteBatch.begin() call, and modify the graphics device settings immediately after, like so:
spriteBatch.Begin(SpriteBlendMode.AlphaBlend,
SpriteSortMode.Immediate, SaveStateMode.None);
graphics.GraphicsDevice.SamplerStates[0].MagFilter = TextureFilter.None;
The key in the spriteBatch.Begin() call is the SpriteSortMode. Setting this value to immediate causes any changes to the graphics device to be applied immediately. And we want to make a change, as you can see in the next line of code. I'm changing the magnification filter to TextureFilter.None because I don't want it to blue anything. After this, you draw and scale your sprites like normal. The result looks like this:
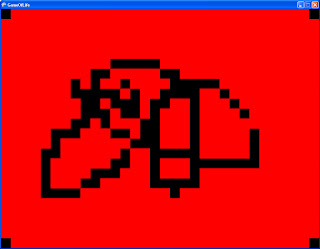
ahh... much better!
1 comment:
Thanks, I've been looking for a soln for this. Just learning XNA after dumping MDX, but I feel like I have to relearn how to do everything. Now just to work out how to actually draw to a bitmap, I'm guessing I have to use .SetData<> and .GetData<>. Thanks.
Post a Comment